Hello everybody,
We started writing about Selenium 4 since last week. Today, I am going to write about one of the most anticipated features of Selenium4. Now Selenium has native support for Chrome DevTools. You can trigger DevTools command during your test.
Other articles about new Selenium 4 features can be found:
Let’s start with DevTools.
What’s Chrome Dev Tools?
Chrome DevTools is a set of web developer tools built directly into the Google Chrome browser. DevTools can help you edit pages on-the-fly and diagnose problems quickly, which ultimately helps you build better websites, faster.
Emulate Network Type
You can emulate different network types by using the below snippet. All you need to do is to change the download/upload throughput and ConnectionType. You can also disconnect your Chrome browser’s connection and run your test by setting the first parameter to true in emulateNetworkConditions method. You can change the values and see how load time changes.
@Test public void emulateNetworkConditionTest(){ chromeDevTools.send(Network.enable(Optional.of(1000000), Optional.empty(), Optional.empty())); chromeDevTools.send( emulateNetworkConditions(false,100,200000,100000, Optional.of(ConnectionType.cellular4g))); long startTime = System.currentTimeMillis(); driver.navigate().to("https://www.swtestacademy.com"); long endTime = System.currentTimeMillis(); System.out.println("Load time is "+ (endTime-startTime)); }
Override UserAgent
The User-Agent request header contains a characteristic string that allows the network protocol peers to identify the application type, operating system, software vendor or software version of the requesting software user agent.
Let’s modify our UserAgent and check if it worked or not. chromeDevTools‘ send method take Network object as a parameter. That object has a method called setUserAgentOverride which takes a string argument. This argument is going to be your new UserAgent string.
@Test public void userAgentOverride(){ String fakeAgent = "Mozilla/5.0 (Windows NT 10.0; WOW64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.81 Safari/537.36"; driver.navigate().to("https://www.whoishostingthis.com/tools/user-agent/"); Assert.assertNotEquals(driver.findElement(By.cssSelector(".info-box.user-agent")).getText(),fakeAgent); chromeDevTools.send(Network.setUserAgentOverride(fakeAgent, Optional.empty(), Optional.empty())); driver.navigate().to("https://www.whoishostingthis.com/tools/user-agent/"); Assert.assertEquals(driver.findElement(By.cssSelector(".info-box.user-agent")).getText(),fakeAgent); }
What does it change? For that test, there is no significant importance. It did not changed anything on the web app.
Sometimes, we want to test mobile web apps and that’s where UserAgent comes into play. We can change our browser to a mobile browser by providing a mobile user agent.
@Test public void webToMobileView(){ String fakeAgent = "Mozilla/5.0 (Linux; Android 7.0; SM-G930V Build/NRD90M) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/59.0.3071.125 Mobile Safari/537.36"; chromeDevTools.send(Network.setUserAgentOverride(fakeAgent, Optional.empty(), Optional.empty())); driver.navigate().to("https://www.amazon.com"); }
Now the web site open in the mobile web view.
Load Insecure Web Site
Sometimes, web sites have SSL issues. You need to approve that you want to continue navigating the web site. However, ChromeDevTools allows you to accept that warning easily.
@Test public void loadInsecureWebsite() { chromeDevTools.send(Security.enable()); chromeDevTools.send(Security.setIgnoreCertificateErrors(true)); driver.get("https://expired.badssl.com/"); }
Now you’ll be able to navigate the page without having any warning page.
Those were some samples that we were able to discover at first glance. We’ll provide more example in near future.
Check out the sample code in that link
Happy testing
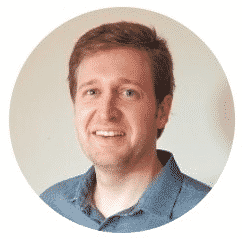
Canberk Akduygu is a Test Lead working in the Netherlands
Thanks for writing this article. Very helpful.
To improve the visibility of the code snippet. You can write this as a GitHub gist and then paste the link.
WordPress would automatically render nicely formatted code for you.
Hi, please can you add more details to capture rest api response etc.
Awesome!
I tried and iked Puppeteer’s capabilities.
I prefer Selenium over all test automation tools so I am really exited to try the Dev Tools Se API.
Thanks!
Thanks, very helpful!
Welcome. :)
Is there any possible way of generating HAR using this new feature