Automation testers are very curious nowadays because Selenium 4 is about to be released, we wanted to show you some sample codes for the new features. There are big changes in Selenium 4 on WebDriver and Selenium Grid parts. In that article we’ll focus on Selenium 4 features below:
- Taking Element Screenshot
- Open New Window and Tab
- Get Element Location and Size
There are many more features coming like Chrome DevTool protocol and Friendly (Relative) Locators. Those are big concepts and we’ll concentrate on them in separate articles.
To add Selenium 4 to your Java project you can use Maven or Gradle.
Maven Dependency
<!-- https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java --> <dependency> <groupId>org.seleniumhq.selenium</groupId> <artifactId>selenium-java</artifactId> <version>4.0.0-alpha-3</version> </dependency>
Gradle Dependency
// https://mvnrepository.com/artifact/org.seleniumhq.selenium/selenium-java compile group: 'org.seleniumhq.selenium', name: 'selenium-java', version: '4.0.0-alpha-3'
Take a Screenshot of a WebElement
WebElement object has a new method called getScreenshotAs which enables you to take a screenshot of a specific WebElement. Here’s the snippet.
@Test public void screenshotTest() throws IOException { WebDriverManager.chromedriver().setup(); WebDriver driver = new ChromeDriver(); driver.navigate().to("https://www.swtestacademy.com"); WebElement logo = driver.findElement(By.className("fusion-logo-link")); File file = logo.getScreenshotAs(OutputType.FILE); FileUtils.copyFile(file,new File("logo.png")); }
Open Window of Tab
You can now open a blank window or a tab on your browser. You can use the below snippet to open a new tab. After opening a new tab, you can use navigate().to() methods to go to another page.
@Test public void openNewTab(){ WebDriverManager.chromedriver().setup(); WebDriver driver = new ChromeDriver(); driver.navigate().to("https://www.google.com"); driver.switchTo().newWindow(WindowType.TAB); driver.navigate().to("https://www.swtestacademy.com"); }
Opening a new window is also very easy. All you need to do is to change the WindowType to WINDOW.
@Test public void openNewWindow(){ WebDriverManager.chromedriver().setup(); WebDriver driver = new ChromeDriver(); driver.navigate().to("https://www.google.com"); driver.switchTo().newWindow(WindowType.WINDOW); driver.navigate().to("https://www.swtestacademy.com"); }
Getting Object Location and Size
In the previous version of Selenium, there was a method to access the Dimension of a WebElement. Now, there’s a Rectangle object. By using this Rectangle property, you can access the location and size of the WebElement.
@Test public void location(){ WebDriverManager.chromedriver().setup(); WebDriver driver = new ChromeDriver(); driver.navigate().to("https://www.swtestacademy.com"); WebElement logo = driver.findElement(By.className("fusion-logo-link")); System.out.println("Height is "+logo.getRect().getDimension().getHeight()); System.out.println("Width is "+logo.getRect().getDimension().getWidth()); System.out.println("Location X is "+logo.getRect().getX()); System.out.println("Location Y is "+logo.getRect().getY()); }
In the next article, we’ll deep dive into the advanced features of Selenium 4.
GitHub Project
https://github.com/swtestacademy/Selenium4
Happy testing,
Canberk Akduygu
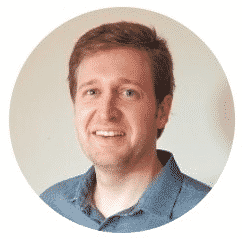
Canberk Akduygu is a Test Lead working in the Netherlands
There is a typo in this section ‘Getting Object Location and Size’, the first time rectangle is used.
thanks for the article!
we already fixed that, thank you.
I could see getRect() in Selenium 3 also, its’s not just available in Selenium 4