JIRA is a very important tool for most IT teams. It is an issue tracking product that allows bug tracking and agile project management. Today, I am going to tell how to integrate JIRA with your test automation project to create automatic bug records.
When I was working as a test consultant, most of the clients would ask the same question. Can we create a ticket to JIRA after a test fails? Of course, we can do it, we can develop any solution. But would that be feasible? Because we might have flaky tests or false-positive results after the test execution. For that reason, I never suggested to those companies implementation of this integration.
But anyway, let’s see how we can create JIRA tickets!
Dependencies
I am using the Jersey-Client library for Base64 transformation and Apache HttpClient libraries.
Here are the Maven Dependencies
<dependency> <groupId>com.sun.jersey</groupId> <artifactId>jersey-client</artifactId> <version>1.8</version> </dependency> <dependency> <groupId>org.apache.httpcomponents</groupId> <artifactId>httpclient</artifactId> <version>4.5.7</version> </dependency>
Authentication
I am going to use Basic Authentication as an example. There are many other ways to authenticate. You can just take a look at the JIRA documentation.
First, we need to create a Base64 key by using the JIRA username and API token.
You can create an API token by using this link.
String auth = new String(Base64.encode("JIRA_USER_NAME" + ":" + "API_TOKEN"));
Your token will be something like this:
Y2FuYmVya2FrZHV5Z3Urc3d0ZXN0YWNhZGVteUBn3WF1b2C5jb206YnhpeFdaT3E5eUtMaGhKZTRyNmdBOT
Payload Example
In order to create a BUG ticket in JIRA, you need to post a JSON payload to the Restful URL.
The basic payload should include the Subject, Project key, and Issue Type. But I also added the description.
{"fields": { "project":{"key":"SWTES"}, "summary":"REST Test", "description":"{code}DB.EXECUTION.ERROR{code} Got 500 as Htp Status Code", "issuetype":{"name":"Bug"} } }
You can basically get the project key by fetching another endpoint. But I am going to hard code it into the JSON file.
Our issue type will be Bug. All JIRA projects have Bug as an issue type. If you have a custom one, you should change this value with a customs value. Summary and Description is a free format. I just use the {code} tag to style my description. I’ll show you the output at the end of the article.
CREATE THE TICKET
Here I am going to apply the HTTP Post Basics that I talked about in that article.
CloseableHttpClient client = HttpClientBuilder.create().build(); HttpPost httpPost = new HttpPost("https://JIRA_URL/rest/api/2/issue"); httpPost.setHeader(HttpHeaders.AUTHORIZATION, "Basic "+auth); httpPost.setHeader(HttpHeaders.CONTENT_TYPE, "application/json"); httpPost.setHeader(HttpHeaders.ACCEPT, "application/json");
String payload is below.
{"fields": { "project":{"key":"SWTES"}, "summary":"REST Test", "description":"{code}DB.EXECUTION.ERROR{code} Got 500 as Htp Status Code", "issuetype":{"name":"Bug"} } }
We post the payload.
StringEntity entity = new StringEntity(body); httpPost.setEntity(entity); HttpResponse response = client.execute(httpPost);
Then we read the response code and response content.
int statusCode = response.getStatusLine().getStatusCode(); String responseBody = EntityUtils.toString(response.getEntity()); System.out.println("Body: " +responseBody); System.out.println("Status Code: " +statusCode);
The response will be something like this.
Body: {"id":"10008","key":"SWTES-9","self":"https://JIRA_URL/rest/api/2/issue/10008"} Status Code: 201
Of course, you should change the architecture of the code before implementing it into your project. Better to parametrize the username, token, etc.
Here’s the output of our ticket:
Source Code can be found in https://github.com/swtestacademy/jiraIntegration
All you need to do is to integrate this code with the Test Runner framework that you use.
Happy Integrations!
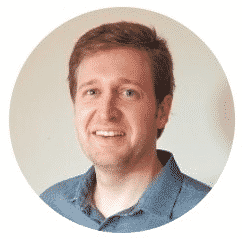
Canberk Akduygu is a Test Lead working in the Netherlands
Just a suggestion. I hope you are aware of this The Jira integration with Selenium covers only more a jira task updation. . Jira zephyr Zapi this is the api for Test case execution integration with Selenium or any Java related api related like ( testcard pass/Fail, test Care and test steps, test case creation . Adding this to the course would add a value to swetestacademy.com
Actually, We just want to show that any integration is possible when you start coding. In that tutorial, we showed how to create a Jira ticket. We have no integration to JUnit or TestNG, it’s just pure Java implementation for JIRA. We have another tutorial to demonstrate “TestRail-Selenium” integration
Nice course!
Hello Canberk Akduygu,
Thanks for your post. Here’s the Creating case. Could you show me How to update an existing ticket on Jira?