This article will show you how to use JSONPath in Gatling tests.
Our sample RESTful web service is https://jsonplaceholder.typicode.com/ and we are going to use /todos endpoint in our tutorial. This endpoint will return a bunch of items, then we’ll fetch the ids’ from the response and make an individual request to /todos endpoint as /todos/{id}.
Define HTTP Protocol
That’s where we define our base url and other configuration like headers, etc. For this example, all we need to have is baseUrl.
object Protocols { val httpProtocol = http .baseUrl("https://jsonplaceholder.typicode.com/") }
Make GET Request to Endpoint
This step is very straight forward. All you need to do is to make a basic GET request.
object Task { def get_task = exec(http("Get all task ids") .get("/todos") }
Extract IDs from Response
You need to use the check function to be able to extract data. Then you can use any jsonpath expression according to your need. After getting the value(s), you should store by using findAll.saveAs() function by giving a variable name.
object Task { def get_task = exec(http("Get All Task Ids") .get("/todos") .check(jsonPath("$[*].id").findAll.saveAs("taskIds"))) }
Make Specific GET Request
Now we have all the ID’s fetch from the response. We create a foreach loop and assign the saved value to another variable and make another get request. In order to call you variable in Scala script, you need to use ${variableName} convention.
object Task { def get_task = exec(http("Get All Task Ids") .get("/todos") .check(jsonPath("$[*].id").findAll.saveAs("taskIds"))) .foreach("${taskIds}", "taskId") { exec(http("Get task info") .get("/todos/${taskId}") )}
Setup Test Configuration
We define our scenario by assigning the test case. Then we configure the virtual user number and ramp up times.
val id_scenario = scenario("Execute Sample API Test").exec(Task.jsonPath) setUp(id_scenario.inject(rampUsers(20) during (10 seconds))).protocols(Protocols.httpProtocol)
Now you can run your Gatling test like a charm.
The whole source code is below
package computerdatabase import scala.concurrent.duration._ import java.util.concurrent.ThreadLocalRandom import io.gatling.core.Predef._ import io.gatling.http.Predef._ class DemoScenario extends Simulation { object Protocols { val httpProtocol = http .baseUrl("http://www.dneonline.com") .contentTypeHeader("text/xml; charset=utf-8").build } object Task { def jsonPath = exec(http("Get all task ids") .get("/todos") .check(jsonPath("$[*].id").findAll.saveAs("taskIds"))) .foreach("${taskIds}", "taskId") { exec(http("Get task info") .get("/todos/${taskId}") )} } val id_scenario = scenario("Execute Sample API Test").exec(Task.jsonPath) setUp(id_scenario.inject(rampUsers(20) during (10 seconds))).protocols(Protocols.httpProtocol) }
Canberk.
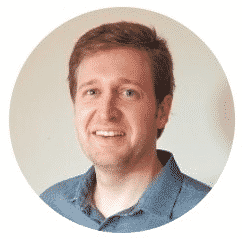
Canberk Akduygu is a Test Lead working in the Netherlands
I have recorded below script. Here we got csrf_token session. Can u please tell me hoe to correlate this
val scn = scenario(“MyFirstGatlingScript”)
// Launch_Page
.exec(http(“request_0”)
.get(“/orangehrm/symfony/web/index.php/auth/login”)
.headers(headers_0))
.pause(15)
// Login
.exec(http(“request_1”)
.post(“/orangehrm/symfony/web/index.php/auth/validateCredentials”)
.headers(headers_1)
.formParam(“actionID”, “”)
.formParam(“hdnUserTimeZoneOffset”, “5.5”)
.formParam(“_csrf_token”, “(.*?)”)
.formParam(“txtUsername”, “Admin”)
.formParam(“txtPassword”, “Admin@123”)
.formParam(“Submit”, “LOGIN”)
.resources(http(“request_2”)
.get(“/orangehrm/symfony/web/index.php/dashboard/employeeDistribution”)
.headers(headers_2),
http(“request_3”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/flot/jquery.flot.min.js?_=1550223416671”)
.headers(headers_3),
http(“request_4”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/flot/jquery.flot.pie.min.js?_=1550223416741”)
.headers(headers_3),
http(“request_5”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/flot/JUMFlot.min.js?_=1550223416763”)
.headers(headers_3),
http(“request_6”)
.get(“/orangehrm/symfony/web/index.php/dashboard/pendingLeaveRequests”)
.headers(headers_2),
http(“request_7”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/graph-visualizer/pie-chart.js?_=1550223416815”)
.headers(headers_3)))
This is the script can u pls tell me how to correlate the session token
import scala.concurrent.duration._
import io.gatling.core.Predef._
import io.gatling.http.Predef._
import io.gatling.jdbc.Predef._
class MyFirstGatlingScript extends Simulation {
val httpProtocol = http
.baseUrl(“http://172.16.203.66:8089”)
.inferHtmlResources()
.acceptHeader(“image/webp,image/apng,image/*,*/*;q=0.8”)
.acceptEncodingHeader(“gzip, deflate”)
.acceptLanguageHeader(“en-US,en;q=0.9”)
.userAgentHeader(“Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/71.0.3578.98 Safari/537.36”)
val headers_0 = Map(
“Accept” -> “text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8”,
“Proxy-Connection” -> “keep-alive”,
“Upgrade-Insecure-Requests” -> “1”)
val headers_1 = Map(
“Accept” -> “text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8”,
“Origin” -> “http://172.16.203.66:8089”,
“Proxy-Connection” -> “keep-alive”,
“Upgrade-Insecure-Requests” -> “1”)
val headers_2 = Map(
“Accept” -> “*/*”,
“Proxy-Connection” -> “keep-alive”,
“X-Requested-With” -> “XMLHttpRequest”)
val headers_3 = Map(
“Accept” -> “text/javascript, application/javascript, application/ecmascript, application/x-ecmascript, */*; q=0.01”,
“Proxy-Connection” -> “keep-alive”,
“X-Requested-With” -> “XMLHttpRequest”)
val headers_8 = Map(“Proxy-Connection” -> “keep-alive”)
val headers_10 = Map(
“Accept” -> “text/css,*/*;q=0.1”,
“Proxy-Connection” -> “keep-alive”)
val headers_11 = Map(
“Accept” -> “*/*”,
“Proxy-Connection” -> “keep-alive”)
val scn = scenario(“MyFirstGatlingScript”)
// Launch_Page
.exec(http(“request_0”)
.get(“/orangehrm/symfony/web/index.php/auth/login”)
.headers(headers_0))
.pause(15)
// Login
.exec(http(“request_1”)
.post(“/orangehrm/symfony/web/index.php/auth/validateCredentials”)
.headers(headers_1)
.formParam(“actionID”, “”)
.formParam(“hdnUserTimeZoneOffset”, “5.5”)
.formParam(“_csrf_token”, “8c8064a0fcfe5a58c3fed3494f5ecf24”)
.formParam(“txtUsername”, “Admin”)
.formParam(“txtPassword”, “Admin@123”)
.formParam(“Submit”, “LOGIN”)
.resources(http(“request_2”)
.get(“/orangehrm/symfony/web/index.php/dashboard/employeeDistribution”)
.headers(headers_2),
http(“request_3”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/flot/jquery.flot.min.js?_=1550223416671”)
.headers(headers_3),
http(“request_4”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/flot/jquery.flot.pie.min.js?_=1550223416741”)
.headers(headers_3),
http(“request_5”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/flot/JUMFlot.min.js?_=1550223416763”)
.headers(headers_3),
http(“request_6”)
.get(“/orangehrm/symfony/web/index.php/dashboard/pendingLeaveRequests”)
.headers(headers_2),
http(“request_7”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmDashboardPlugin/js/graph-visualizer/pie-chart.js?_=1550223416815”)
.headers(headers_3)))
.pause(52)
// Click_Admin
.exec(http(“request_8”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/themes/default/images/top-nav-selected1.png”)
.headers(headers_8)
.resources(http(“request_9”)
.get(“/orangehrm/symfony/web/index.php/admin/viewAdminModule”)
.headers(headers_0),
http(“request_10”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmCorePlugin/css/_ohrmList.css”)
.headers(headers_10),
http(“request_11”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmCorePlugin/js/_ohrmList.js”)
.headers(headers_11),
http(“request_12”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/orangehrmAdminPlugin/js/viewSystemUserSuccess.js”)
.headers(headers_11),
http(“request_13”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/themes/default/images/sub-nav-arrow.png”)
.headers(headers_8),
http(“request_14”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/themes/default/images/tablesorter-bg.png”)
.headers(headers_8),
http(“request_15”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/themes/default/images/toggle-down.png”)
.headers(headers_8),
http(“request_16”)
.get(“/orangehrm/symfony/web/webres_57eb84702e3066.16112222/themes/default/images/sub-nav-arrow-s.png”)
.headers(headers_8)))
.pause(11)
// Logout
.exec(http(“request_17”)
.get(“/orangehrm/symfony/web/index.php/auth/logout”)
.headers(headers_0))
setUp(scn.inject(atOnceUsers(1))).protocols(httpProtocol)
}