In this article, we will learn Primitive Type Streams in Java with examples. I will also show various primitive type conversions in a stream. Let’s start!
Primitive Type Streams in Java
I will explain the primitive types with an example. In the example below, we will do a sum operation and also do several primitive type conversions. We have Stream<Integer> and we will convert it to LongStream, then DoubleStream, and then IntStream. Because of these conversions, the performance of the stream becomes slower. We can also compare this in the Stream Reduce article.
@TestMethodOrder(MethodOrderer.OrderAnnotation.class) public class PrimitiveStreams { List<Integer> numbers = new ArrayList<>(); @BeforeEach void setup() { Collections.addAll(numbers, 1, 2, 3, 4); } @Test @Order(1) public void primitiveStreamConversionTest() { Instant start = Instant.now(); int sum = numbers.stream() .mapToLong(a -> a) .mapToDouble(a -> (double) a) .mapToInt(a -> (int) a) .sum(); System.out.println("Sum of primitiveStreamConversionTest operation: " + sum); Instant end = Instant.now(); System.out.println("Elapsed time of primitiveStreamConversionTest: " + Duration.between(start, end).toNanos()); } }
Output
IntStream Range Examples in Java
In the examples below, I will show the IntStream range behavior. If the range is closed, the stream will use the first and the last of the elements. If the range is not closed, the last of the element is ignored. The range method is (inclusive, exclusive) and the rangeClosed method is (inclusive, inclusive).
@Test @Order(2) public void intStreamRangeTest() { Instant start = Instant.now(); int sum = IntStream.range(1, 3) .sum(); System.out.println("Sum of intStreamRangeTest operation: " + sum); Instant end = Instant.now(); System.out.println("Elapsed time of intStreamRangeTest: " + Duration.between(start, end).toNanos()); } @Test @Order(3) public void intStreamRangeClosedTest() { Instant start = Instant.now(); int sum = IntStream.rangeClosed(1, 4) .sum(); System.out.println("Sum of intStreamRangeClosedTest operation: " + sum); Instant end = Instant.now(); System.out.println("Elapsed time of intStreamRangeClosedTest: " + Duration.between(start, end).toNanos()); }
Output
GitHub Project
In this article, we learned the primitive streams examples in java. See you in the next article!
Thanks for reading,
Onur Baskirt
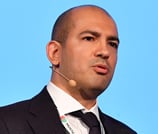
Onur Baskirt is a Software Engineering Leader with international experience in world-class companies. Now, he is a Software Engineering Lead at Emirates Airlines in Dubai.