In this article, you will learn Java 9 functional programming features. These are, takeWhile(), dropWhile(), immutableList, immutableSet, and immutableMap. I will explain each feature with clear examples. Let’s start! :)
Java 9 Functional Programming Features
We will start with takeWhile() method and then continue with the other ones.
TakeWhile()
The stream processes the elements until the condition satisfies, then stops processing.
Official description: Independent of whether this stream is ordered or unordered if all elements of this stream match the given predicate then this operation takes all elements (the result is the same as the input), or if no elements of the stream match the given predicate then no elements are taken (the result is an empty stream).
In the example below, we have a list of numbers from 1 to 10, and by using the takeWhile() method the code processes the elements which are smaller than 6, and when the stream processing comes to 6 it ends the processing.
@TestMethodOrder(MethodOrderer.OrderAnnotation.class) public class Java9Features { List<Integer> numbers = new ArrayList<>(); @BeforeEach public void setup(TestInfo testInfo) { System.out.println(testInfo.getDisplayName()); Collections.addAll(numbers, 1, 2, 4, 5, 7, 6, 8, 3, 9, 10); } @AfterEach public void tearDown() { numbers.clear(); } /** * TakeWhile: It processes the elements until the condition satisfies. Then stops processing. * That's why it does not process 3 even it is smaller than 6. */ @Test @Order(1) public void takeWhileExample() { numbers.stream() .takeWhile(numbers -> numbers < 6) //Prints: 1 2 4 5 .forEach(System.out::println); } }
Output
DropWhile()
It does not process the elements which do not satisfy the condition while it reaches the condition, after this point regardless of the condition it processes the elements.
Official description: Independent of whether this stream is ordered or unordered if all elements of this stream match the given predicate then this operation drops all elements (the result is an empty stream), or if no elements of the stream match the given predicate then no elements are dropped (the result is the same as the input).
In the example below, we have a list of numbers and by using the dropWhile() method, we don’t process elements that are smaller than 6 until we reach the number 6. After that, it starts to process the other elements regardless of condition. That’s why it processes 3 even it is smaller than 6.
/** * DropWhile: It does not process the elements which do not satisfy the condition. * Then starts to process the other elements regardless of conditions. That's why it processes 3 even it is smaller than 6. */ @Test @Order(2) public void dropWhileExample() { numbers.stream() .dropWhile(numbers -> numbers < 6) //Prints: 7 6 8 3 9 10 .forEach(System.out::println); }
Output
Immutable List
List.of() declaration creates a list which is an immutable (constant) list. We cannot modify this list after creation. Let’s see this in the example below.
We created an immutable list below and then tried to add a value to it and got UnsupportedOperationException exception.
/** * Immutable List: List.of() declaration creates a list which is an immutable (constant) list. */ @Test @Order(3) public void immutableList() { List<Integer> listOf = List.of(1, 2, 3, 4, 5, 6); System.out.println(listOf); //List.of() creates immutable (constant) list. Assertions.assertThrows(UnsupportedOperationException.class, () -> listOf.add(7)); }
Output
Immutable Set
Set.of() declaration creates a set which is an immutable (constant) set. We cannot modify this set after creation. Let’s see this in the example below. Note: Sets do not contain multiple elements.
We created an immutable set below and then tried to add a value to it and got UnsupportedOperationException exception.
/** * Immutable Set: Set.of() declaration creates a set which is an immutable (constant) set. */ @Test @Order(4) public void immutableSet() { Set<Integer> setOf = Set.of(1, 2, 3, 4, 5, 6); System.out.println(setOf); //Note: Set does not maintain the order. //Set.of() creates immutable (constant) set. Assertions.assertThrows(UnsupportedOperationException.class, () -> setOf.add(7)); }
Output
Immutable Map
Map.of() declaration creates a map which is an immutable (constant) map. We cannot modify this map after creation. Let’s see this in the example below.
We created an immutable map below and then tried to add a value to it and got UnsupportedOperationException exception.
/** * Immutable Map: Map.of() declaration creates a map which is an immutable (constant) map. */ @Test @Order(5) public void immutableMap() { Map<Integer, String> mapOf = Map.of(1, "Ronaldo", 2, "Messi"); System.out.println(mapOf); //Map.of() creates immutable (constant) map. Assertions.assertThrows(UnsupportedOperationException.class, () -> mapOf.put(3, "Ozil")); }
Output
GitHub Project
In this article, we learned the Java 9 functional programming features and innovations such as takeWhile, dropWhile, immutableList, immutableSet, and immutableMap.
I hope you enjoyed reading it.
Onur Baskirt
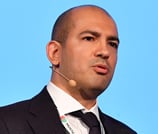
Onur Baskirt is a Software Engineering Leader with international experience in world-class companies. Now, he is a Software Engineering Lead at Emirates Airlines in Dubai.